You might find yourself in a situation where you must remap a value from one range to another.
Let´s say you have the value 50 in the range of 0 to 100 and want to remap the value between 0 and 1, equaling 0.5.
If you are using the Unity Mathematics API, you have that functionality and can use the remap method.
If you need to implement your own remap functionality, you must consider whether the value needs to be clamped or unclamped, meaning if the remapped value can exceed the upper range limit.
Example:
You have the value 110 in the range between 0 and 100 and need to remap it to the range of 0 to 1. Should the maximum outcome be one, or can it go higher?Let´s say you need to remap the value of current hit points in the range from 0 to 600 to a health bar with a range between 0 and 100.
Let´s start by implementing an unclamped method without using any built-in functionality from Unity.
Our method needs to know the value to remap and the old and new range giving us five parameters for our method. We then scale the value to the new range.
private float RemapValueToRange(float value, float oldRangeMin, float oldRangeMax, float newRangeMin, float newRangeMax) { return newRangeMin + (value - oldRangeMin) * (newRangeMax - newRangeMin) / (oldRangeMax - oldRangeMin); }
To implement the clamped version using unity functionality, we perform an inverse lerp of the value using the old range. We then proceed to lerp the result within the new range.
private float RemapValueToRangeWithUnity(float value, float oldRangeMin, float oldRangeMax, float newRangeMin,float newRangeMax) { float normal = Mathf.InverseLerp(oldRangeMin, oldRangeMax, value); return Mathf.Lerp(newRangeMin, newRangeMax, normal); }
If you would like to know a little bit more, you can watch the talk from Freya Holmér at IndieCade Europe.
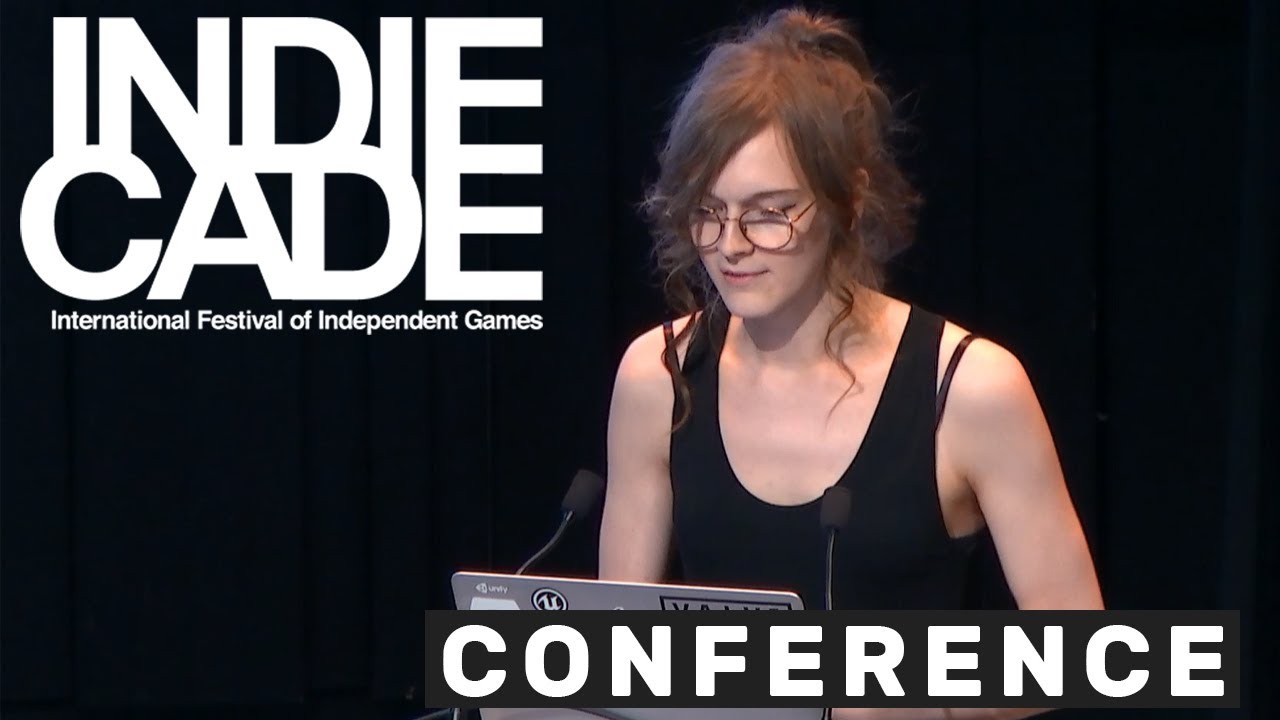